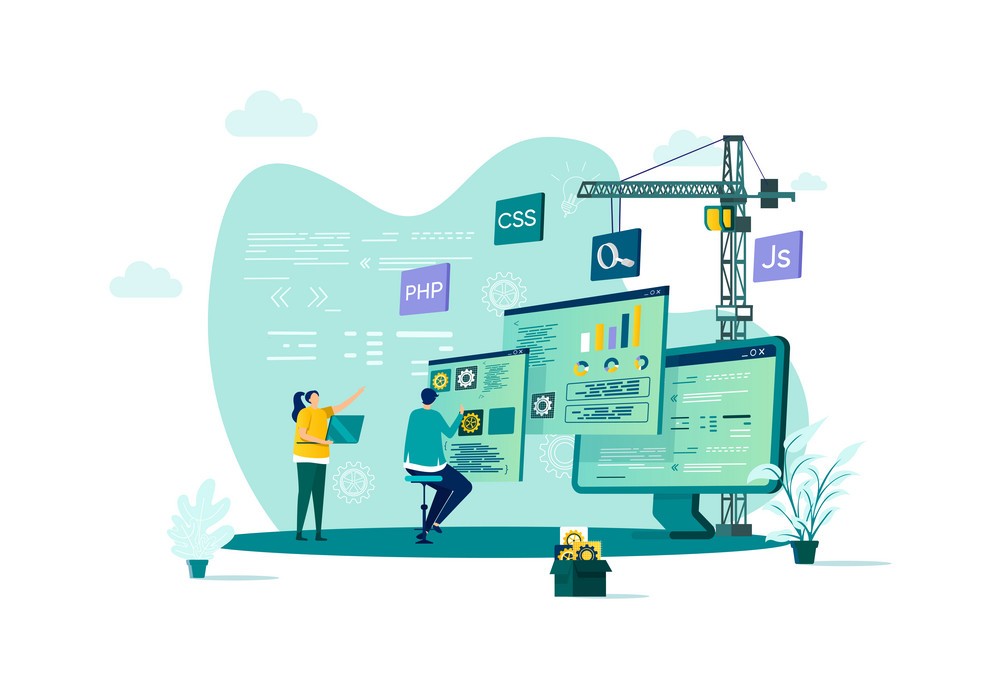
FULL STACK JAVA DEVELOPER
CERTIFICATE PROGRAM IN FULL-STACK JAVA DEVELOPER
FUNDAMENTALS OF SYSTEM ANALYSIS, DESIGN & PROGRAMMING
- IT Fundamental
- Programming Logics and Techniques
- Introduction to OOP (Object-Oriented Programming)
- Data structure and algorithms
- Recursion
- Arrays
- Searching Algorithms
- Sorting Algorithms
- Linked List
- Stacks
- Queues
- Introduction to GitHub and Version Control System
INTRODUCTION TO WEB DEVELOPMENT
- Network and Web Designing Elements
- Types of Networks and Web Programming
- Basic Concepts of Network Models and Web
- Networks and Websites in Real-world
- Technologies and Tools Used in Web Development
- Introduction to Web Services
- Introduction to interactive and responsive web pages
INTRODUCTION TO HTML
- HTML Basics
- Structure and Elements of an HTML Document
- Writing Paragraph on a Webpage
- Styling HTML
- CREATING LISTS AND LINKS IN HTML
- Creating Table in HTML
- Creating Form in HTML
- HTML Form Controls
- Adding Media in Webpage
- Designing with CSS
CASCADING STYLE SHEET (CSS)
- Introduction CSS3
- Types of CSS Selectors
- Creating Cascading Styles
- CSS Borders
- CSS Padding
- CSS Height/Width
- CSS Gradients
- CSS Shadows
- CSS Text/Fonts
- CSS 2D Transforms
- CSS 3D Transform
- CSS Links
- CSS Lists
- CSS Tables
- CSS Box Model
- CSS Outline
- CSS Display
- CSS Max-width
- CSS Position
- CSS Float
- CSS Inline-block
- CSS Align
- CSS Combinators
- CSS Pseudo-class
- CSS Pseudo-element
- CSS Navigation Bar
- CSS Dropdowns
- CSS Tooltips
- CSS Images
- CSS Forms
- CSS Counters
- ANIMATING WITH CSS
- Building blocks of CSS Animation
- CSS Buttons
- CSS Pagination
- CSS Multiple Columns
- CSS User Interface
- CSS Box Sizing
- CSS Filters
- CSS Responsive
BOOTSTRAP
- Introduction to Bootstrap
- Bootstrap Basics
- Bootstrap Grids
- Bootstrap Themes
- Bootstrap CSS
- Bootstrap JS
JAVASCRIPT AND SERVER-SIDE COMMUNICATION
- Introduction to JavaScript
- JavaScript Language Basics
- JavaScript Objects
- JavaScript Scope
- JavaScript Events
- JavaScript Strings
- JavaScript Numbers
- JavaScript Math
- JavaScript Arrays
- JavaScript Boolean
- JavaScript Comparisons
- JavaScript Conditions
- JavaScript Switch
- JavaScript Loops
- JavaScript Type Conversion
- JavaScript RegExp
- JavaScript Errors
- JavaScript Debugging
- JavaScript Hoisting
- JavaScript Strict Mode
- JavaScript Functions
- JavaScript Objects
- JavaScript Forms
- JavaScript HTML DOM
- JavaScript BOM
JQUERY
- Introduction to JSON and jQuery
- jQuery Syntax
- jQuery Selectors
- jQuery Event
- jQuery Effects
- jQuery HTML
- jQuery Traversing
- AJAX Concepts
- AJAX XMLHttpRequest object
- Programming AJAX
- Working with JSON
- jQuery AJAX & Misc
- Making an AJAX call and retrieving the response
- AJAX & API
- Security and Authorization using AJAX
CORE JAVA
- Introduction to Java
- Java Run-Time Environment
- Conditional Statement
- Flow Control Statement
- Data Type
- Arrays
- Functions
- Object-Oriented Programming
- Object-Oriented Programming – Abstraction
- Object-Oriented Programming – Encapsulation
- Object-Oriented Programming – Inheritance
- Object-Oriented Programming – Polymorphism
- String Manipulation
- Packages and Interfaces
- Multi-Threading
- Exception and Error Handling
- Input Output Streams
- Networking in Java
- Wrapper Class
- The Collection Framework
- Inner Classes
- The Abstract Windowing Toolkit Package
- Java Swing
ADVANCE JAVA
- Java Socket
- Java Swing
- Java Swing App
- Java Applet
- Java Servlets
- Java JDBC
- Java Client – Server
- Java Server Page
- Java Datagram Socket
- Java Datagram Packet
- Nonblocking I/O
- Java Mail API
- Java Beans
- Introduction to XML
- Writing XML files
- DOM Parser – Writing into an XML file and Parsing an XML file
- SAX Parser, XSL
- Common Gateway Interface
- JSP Expression Language
- JSP Standard Tag Library
- Filters in Web Application
- Web Application Security
SPRING
- Introduction to Spring Framework
- Architecture
- Display a Sample Message
- IoC Containers
- Bean Definition
- Bean Scopes
- Bean Post Processors
- Dependency Injection Auto-Wiring
HIBERNATE
- Introduction to Hibernate
- Architecture of Hibernate
- Database Operations: Insert/Update/Delete/Select
- Inheritance
- Collections
- HQL and Restrictions
- Caching in Hibernate
MYSQL
MySQL Data Definition using SQL
- Manage Databases
- MySQL Table Types
- MySQL Data Types
- MySQL Create Table
- MySQL Primary Key
- MySQL Foreign Key
- MySQL Sequence
- MySQL INT Data Type
- MySQL Decimal Data Type
- MySQL Date Data Type
- MySQL Time Data Type
- MySQL Datetime Data Type
- MySQL Alter Table
- MySQL Rename Table
- MySQL Add Column
- MySQL Drop Table
- MySQL Temporary Table
Basic Data Manipulation using SQL
- SQL Syntax
- What are DDL, DML, and DCL?
- SQL Select
- SQL Distinct
- SQL Where
- SQL And & Or
- SQL Order By
- SQL Insert Into
- SQL Update
- SQL Delete
- SQL Injection
- SQL Select Top
- SQL Like
- SQL Wildcards
- SQL In
- SQL Between
- SQL Aliases
- SQL Joins
- SQL Inner Join
- SQL Left Join
- SQL Right Join
- SQL Full Join
- SQL Union
- SQL Select Into
- SQL Insert Into Select
- SQL Create DB
- SQL Create Table
- SQL Constraints
- SQL Not Null
- SQL Unique
- SQL Primary Key
- SQL Foreign Key
- SQL Check
- SQL Default
- SQL Create Index
- SQL Drop
- SQL Alter
- SQL Auto Increment
- SQL Views
- SQL Dates
- SQL Null Values
- SQL Null Functions
- SQL Data Types
- SQL DB Data Types
SQL Functions
- SQL Avg()
- SQL Count()
- SQL First()
- SQL Last()
- SQL Max()
- SQL Min()
- SQL Sum()
- SQL Group By
- SQL Having
- SQL Ucase()
- SQL Lcase()
- SQL Mid()
- SQL Len()
- SQL Round()
- SQL Now()
- SQL Format()
MySQL Views
- Introduction to Database View
- Views in MySQL
- MySQL Create View
- MySQL Views & With Check Option
- Creating MySQL Updatable Views
- Local & Cascaded in With Check Option
- Managing Views in MySQL
ANGULAR.JS
- Introduction to Angular
- Comparison between front-end tools
- Angular Architecture
- Building blocks of Angular
- Angular Installation
- Angular CLI
- Angular CLI commands
- Angular Modules
- Understanding files in Angular
- Installation of Node.js, Angular CLI, and Visual Studio Code
- Creating First Angular Application
Angular Components and Data Binding
- Working on Angular Applications
- Angular App Bootstrapping
- Angular Components
- Creating A Component Through Angular CLI
- Ways to specify selectors
- Template and styles
- Installing bootstrap to design application
- Data Binding
- Types of Data Binding
- Component Interaction using @Input and @Output decorator
- Angular Animations
- Component Life-cycle Hooks
Directives and Pipes in Angular
- Understanding Angular Directives
- @Component Directive
- Structural Directives
- Attribute Directives
- Custom Directives
- Pipes
- Built-in Pipes
- Chaining pipes
- Custom pipes
- Pipe Transform Interface & Transform Function
- Pure and Impure pipes
Angular Services and Dependency Injection
- Angular service
- Need for a service
- Dependency Injection
- Creating a service
- Hierarchical Injector
- Injecting A Service into Another Service
- Observables
- RxJS Library
- Angular’s Interaction with Backend
- Parts of an HTTP Request
- HttpClient
Angular Routes and Navigation
- Angular Router
- Setting Up Routes
- Adding Routes Using Router Link
- Wildcard and Redirecting Routes
- Adding Navigation Programmatically
- Passing Route Parameters
- Extracting Parameters Using Activated Route
- Optional Route Parameters
- Child Routes
- Route Guards
- Location Strategies
- Build a server management application and make use of routing
- Make use of route guards to prevent navigation to different pages
Handling Forms in Angular
- Angular forms
- Types of forms
- Underlying building blocks of the form model
- Template-driven vs Reactive forms
- Template-driven forms
- Reactive Forms
- Dynamically adding data to a form
- Build a user registration form using a template-driven approach
- Build a user registration form using a reactive approach
Validating Angular Forms
- What is Form Validation?
- Types of Form Validation
- Built-in Validators
- Form control’s status and validity
- Form Validation methods
- CSS classes for the Form control
- Custom validators in Template Driven Forms
Authentication with JWT and Security in Angular
- What is Authentication?
- Authentication and authorization
- Types of Authentications
- Where to store tokens?
- JSON Web Tokens (JWT)
- Authentication in Angular application
- Security threats in web application
- Create Login and registration form and store user data using fake-backend provider
- Authenticate a user using JWT authentication for login form
Application Deployment in Angular
- Running application on the production server: Nginx
- Architecture of Nginx
- How to configure Nginx?
- Deployment of an application using Docker
- Problems before containers
- How containers solve the problems
- What is Docker?
- Docker file
- Docker image
- Docker containers
- Docker hub
- Basic Docker commands
- Testing Angular application
- Application Deployment via Docker