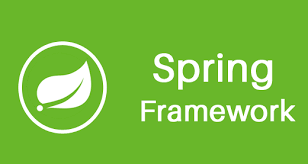
Spring 5.x Internship
The Spring Framework is
an open source application framework for Java. This framework has taken the
Java software community by storm. Spring provided the technology to develop
everything from small, stand-alone applications to large complex, enterprise systems
out of simple POJOs (plain old Java objects).
In this class, students
are exposed to the light-weight Spring container, configuration, foundational
API, and general Spring architecture. Not just a class that focuses on theory,
this course is loaded with practical labs and deals with configuration,
maintenance and architectural issues. After taking the class, developers will
immediately be able to utilize the Spring Framework in their new or existing
applications.
Spring 5.x Course Prerequisite
- Students should have a good understanding
of the Java programming language. A basic understanding of relational
databases and SQL is very helpful. A basic understanding of XML is also
useful. Students that have attended Intertech’s Complete Java have
the necessary background for this course.
Spring 5.x Training Course Objective
- Learn how to download, setup and configure
the Spring Framework
- Explore the Spring Container and Modules
- Discover the Spring philosophies and
principles and how they impact application development
- Understand dependency injection
- Learn aspect oriented programming and how
it is used to provide cross cutting concerns
- See how to accomplish data access with
Spring’s DAO Module
- Understand how Spring deals with transaction
management
- Examine Spring’s unit testing framework
Spring 5.x Training Course Duration
- 60 Working days, daily one and half hours
Spring 5.x Training Overview
Spring Introduction
- Differences between programming language,
software technology and framework
- Introduction to Spring Framework
- Evolution of spring Framework
- Modules of Spring in Spring 1.x,2.x,3.x,4.x
and 5.x
- MVC Architecture
- Role of spring framework in MVC
Architecture application development
- Definition of spring framework
- POJO Class, POJI, JavaBean, Component Class
,spring bean classes
Spring Core Module
- Introduction to IOC
- Introduction to Spring Container/IOC
Container
- Types of Dependency Injections
· Setter injection
· Constructor injection
· Aware injection
· Method injection
· Lookup method injection
- Introduction to Design patterns
- Factory DesignPattern
- Strategy Design pattern
- Layered Application demonstrating real time
dependency injection
- Resolving/identifying params in constructor
injection
- Bean Inheritance
- Collection Merging
- Null injections
- Bean alias
- Default bean ids
- Performing dependency lookup by using IOC
container
- <Idref>tag
- Understanding Factory Methods
- Factory Method Bean Instantiation
· Static factory method bean Instantiation
· instance factory method bean Instantiation
- Singleton java class and its usecases
- Bean Scopes
· Singleton
· Prototype
· Request
· Session
· Application
· Websocket
- Bean Wiring
· Explicit wiring
· Implicit wiring or auto wiring
· ByType
· ByName
· Constructor
· Autodetect
- p-namespace,C-namespace
- ApplicationContext Container
- Preinstantiation of singleton scope beans
- Working with properties file
- I18n (Internationalization)
- Event Handling
- BeanFactory Vs ApplicationContext
- Automatic registration of bean post
processor and bean factory post processor
- Bean Life Cycle
- Declarative approach
- Programmatic approach
- Annotation driven approach
- Nested IOC Containers
- Presentation tier
- Business tier
- Various attributes of <ref> tag
- FactoryBean
- ServiceLocator as factory bean
- FactoryMethod bean Instantation based
service locator
- Method Replacement/Method Injection
- Aware Injection
- Lookup method injection
- BeanPostProcessor
- BeanFactoryPostProcessors
- PropertyEditors
- Custom property editors
- Spring Expression Language(SpEL)
Spring Core Module with Annotations
- Spring stereo type annotations
- @Component,@Service,@controller,@Repositry
and etc…
- @Autowired,@Qualifier,@Lazy and etc…
- Working with Java config annotations
- @Named,@Inject,@Resource and etc…
- Working with properties file in annotations
environment
- Developing Layered applications in
annotations environment
Spring Core Module with 100% Code/Java Config Approach.
- Working with @Bean,
@Configuration,@Lazy,@PropertySource and etc…
- Developing Layered application
- Working with
AnnotationConfigApplicationContext
Spring Boot Core
- Introduction to Spring Boot
- Spring Boot primary goals
- Spring boot features
- Spring Boot Starters
- Understanding @SpringBootApplication
- Auto configuration
- Example Applications
- Spring Profiles
- properties Vs application.yml
- Spring Boot Standalone flow
- Working with sts plugins in eclipse to
develop spring boot application
- Spring stereo type annotations
Spring JDBC/DAO
- Introduction
- Plain JDBC limitations
- Spring JDBC/DAO Advantages
- Working with different Data Sources
- JdbcTemplate
- JNDI Registry and ServerManaged Jdbc
connection pool
- Introduction
- Differences between programming language,
software technology and framework